今回は「指定された期間のGoogleカレンダー上の予定を別日に移動させるスクリプト」について、GASのコードの紹介と各処理の簡単な解説をしていく。
今までに作成したGASのスクリプトの一覧はこちら。
作成するスクリプトの概要と使いどころ
「指定された期間のGoogleカレンダー上の予定を別日に移動させるスクリプト」についての概要は以下の通り。
・Googleカレンダー上の予定を期間(複数日)で指定してすべて取得する
・タイトルに特定のキーワードを含んだ予定は移動対象から除外する
・「移動対象の期間」「移動先の日付」「除外するキーワード」はスプレッドシート内の特定のセルから取得できるようにする
コメントなどを除けばおよそ100行ほどのシンプルな処理のスクリプトで、以下のようにスプレッドシート上にメイン関数を割り当てたボタンを用意しつつ必要な情報を入力してあげれば、ボタン1つで簡単に使い始めることができる。
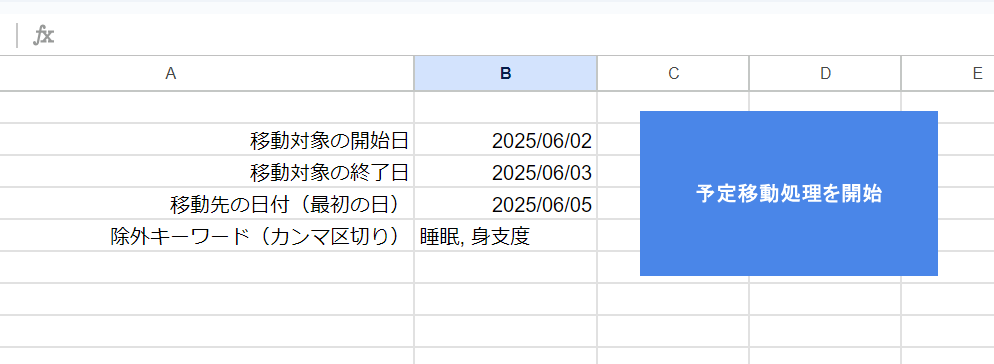
このスクリプトの使いどころとして、例えば「一か月後に旅行の計画を立ててGoogleカレンダーにも予定を入れていたが、旅行の日程が変わってしまった」といったような「数日分の予定をまとめて移動したいとき」なんかに意外と重宝する。
コードの紹介と各処理の簡単な解説【コピペOK】
今回のGASスクリプトのコードは以下の通り。
こちらのコードに関しては特に変更を加えなくても、皆さん自身の任意のスプレッドシート上に必要な情報を入力し、コンテナバインドスクリプトとして動かすことでそのまま使用することができる。
/**
* Google Calendar上の予定を指定期間から別の日付に移動するスクリプト
* - 予定を期間(複数日)で指定して移動
* - タイトルに特定のキーワードを含む予定は除外
* - 移動対象期間、移動先日付、除外キーワードはスプレッドシートから取得
*/
// メイン関数
function moveCalendarEvents() {
try {
// スプレッドシートから設定値を取得
const settings = getSettings();
// 対象期間の予定を取得
const events = fetchEvents(settings.startDate, settings.endDate, settings.excludeKeywords);
// 予定を移動
const movedCount = moveEvents(events, settings.daysDifference);
// 結果をログ出力
console.log(`移動完了: ${movedCount}件の予定を移動しました。`);
} catch (error) {
console.log(`エラーが発生しました: ${error.message}`);
}
}
/**
* スプレッドシートから設定値を取得する
* @return {Object} 設定値のオブジェクト
*/
function getSettings() {
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("設定");
// 各設定値の取得
const startDateValue = sheet.getRange("B2").getValue(); // 移動対象の開始日
const endDateValue = sheet.getRange("B3").getValue(); // 移動対象の終了日
const targetDateValue = sheet.getRange("B4").getValue(); // 移動先の日付(最初の日)
const excludeKeywordsValue = sheet.getRange("B5").getValue(); // 除外キーワード(カンマ区切りで複数指定可能)
// 日付のバリデーション
if (
!(startDateValue instanceof Date) ||
!(endDateValue instanceof Date) ||
!(targetDateValue instanceof Date)
) {
throw new Error("日付の形式が正しくありません");
}
// 終了日を1日後の0時に設定
const adjustedEndDate = new Date(endDateValue);
adjustedEndDate.setDate(endDateValue.getDate() + 1);
// 除外キーワードの処理(カンマ区切りでキーワードを指定可能に)
const excludeKeywords = excludeKeywordsValue ? excludeKeywordsValue.split(",").map((keyword) => keyword.trim()) : [];
// 移動日数の計算(対象期間の開始日と移動先日付の差)
const daysDifference = Math.floor((targetDateValue - startDateValue) / (1000 * 60 * 60 * 24));
return {
startDate: startDateValue,
endDate: adjustedEndDate,
daysDifference: daysDifference,
excludeKeywords: excludeKeywords,
};
}
/**
* 指定期間内の予定を取得する(除外キーワードを含む予定は除外)
* @param {Date} startDate - 開始日
* @param {Date} endDate - 終了日
* @param {Array} excludeKeywords - 除外キーワードのリスト
* @return {Array} 取得した予定の配列
*/
function fetchEvents(startDate, endDate, excludeKeywords) {
// デフォルトカレンダーを取得
const calendar = CalendarApp.getDefaultCalendar();
// 指定期間の予定を取得
const allEvents = calendar.getEvents(startDate, endDate);
// 除外キーワードを含まない予定だけをフィルタリング
const filteredEvents = allEvents.filter((event) => {
const title = event.getTitle();
return !excludeKeywords.some((keyword) => title.includes(keyword));
});
return filteredEvents;
}
/**
* 予定を指定した日数分移動する
* @param {Array} events - 移動する予定の配列
* @param {number} daysDifference - 移動する日数
* @return {Object} 移動結果の統計
*/
function moveEvents(events, daysDifference) {
let movedCount = 0;
// 各予定を移動
events.forEach((event) => {
// 開始時刻と終了時刻を取得
const startTime = event.getStartTime();
const endTime = event.getEndTime();
// 新しい日時を計算(時刻はそのまま、日付だけ移動)
const newStartTime = new Date(startTime);
newStartTime.setDate(startTime.getDate() + daysDifference);
const newEndTime = new Date(endTime);
newEndTime.setDate(endTime.getDate() + daysDifference);
// 予定を移動
event.setTime(newStartTime, newEndTime);
movedCount++;
});
return movedCount;
}
以降の章では、上記のコードについて関数ごとに処理の概要を簡単に解説していく。
moveCalendarEvents 関数
/**
* Google Calendar上の予定を指定期間から別の日付に移動するスクリプト
* - 予定を期間(複数日)で指定して移動
* - タイトルに特定のキーワードを含む予定は除外
* - 移動対象期間、移動先日付、除外キーワードはスプレッドシートから取得
*/
// メイン関数
function moveCalendarEvents() {
try {
// スプレッドシートから設定値を取得
const settings = getSettings();
// 対象期間の予定を取得
const events = fetchEvents(settings.startDate, settings.endDate, settings.excludeKeywords);
// 予定を移動
const movedCount = moveEvents(events, settings.daysDifference);
// 結果をログ出力
console.log(`移動完了: ${movedCount}件の予定を移動しました。`);
} catch (error) {
console.log(`エラーが発生しました: ${error.message}`);
}
}
メイン実行用の関数で、ここから以下の関数を実行している。
・getSettings 関数:スプレッドシートから「移動対象の期間」「移動先の日付」「除外するキーワード」を取得する関数
・fetchEvents 関数:getSettings 関数で取得した情報をもとに、移動対象の予定をGoogleカレンダー上から取得する関数
・moveEvents 関数:実際にGoogleカレンダー上で予定の移動処理を行う関数
関数内の処理は「try-catch」文で囲み、処理中に何かしらのエラーになった場合にはコンソールにログを出力するようにしている。
また各関数の処理が正常に行われた場合には、実際に移動された予定の件数もコンソールに出力する。
getSettings 関数
/**
* スプレッドシートから設定値を取得する
* @return {Object} 設定値のオブジェクト
*/
function getSettings() {
const sheet = SpreadsheetApp.getActiveSpreadsheet().getSheetByName("設定");
// 各設定値の取得
const startDateValue = sheet.getRange("B2").getValue(); // 移動対象の開始日
const endDateValue = sheet.getRange("B3").getValue(); // 移動対象の終了日
const targetDateValue = sheet.getRange("B4").getValue(); // 移動先の日付(最初の日)
const excludeKeywordsValue = sheet.getRange("B5").getValue(); // 除外キーワード(カンマ区切りで複数指定可能)
// 日付のバリデーション
if (
!(startDateValue instanceof Date) ||
!(endDateValue instanceof Date) ||
!(targetDateValue instanceof Date)
) {
throw new Error("日付の形式が正しくありません");
}
// 終了日を1日後の0時に設定
const adjustedEndDate = new Date(endDateValue);
adjustedEndDate.setDate(endDateValue.getDate() + 1);
// 除外キーワードの処理(カンマ区切りでキーワードを指定可能に)
const excludeKeywords = excludeKeywordsValue ? excludeKeywordsValue.split(",").map((keyword) => keyword.trim()) : [];
// 移動日数の計算(対象期間の開始日と移動先日付の差)
const daysDifference = Math.floor((targetDateValue - startDateValue) / (1000 * 60 * 60 * 24));
return {
startDate: startDateValue,
endDate: adjustedEndDate,
daysDifference: daysDifference,
excludeKeywords: excludeKeywords,
};
}
メイン関数から一番最初に呼ばれる関数で、スプレッドシートの特定のセルから以下の4つの値を取得している。
・移動対象の開始日:移動元の予定がある期間の最初の日。今回のコードでは「B2」セルから取得
・移動対象の終了日:移動元の予定がある期間の最後の日。今回のコードでは「B3」セルから取得
・移動先の日付:予定を移動先の最初の日。今回のコードでは「B4」セルから取得
・除外キーワード:予定を移動対象から除外するためのキーワード。今回のコードでは「B5」セルから取得
冒頭の画像の再掲となるが、スプレッドシート上では以下のようなイメージ。
この例では、「『睡眠』『身支度』というキーワードをタイトルに含む予定を除き、『2025/6/2~6/3』の2日分の予定を『2025/6/5~6/6』の期間へ移動する」という処理となる。
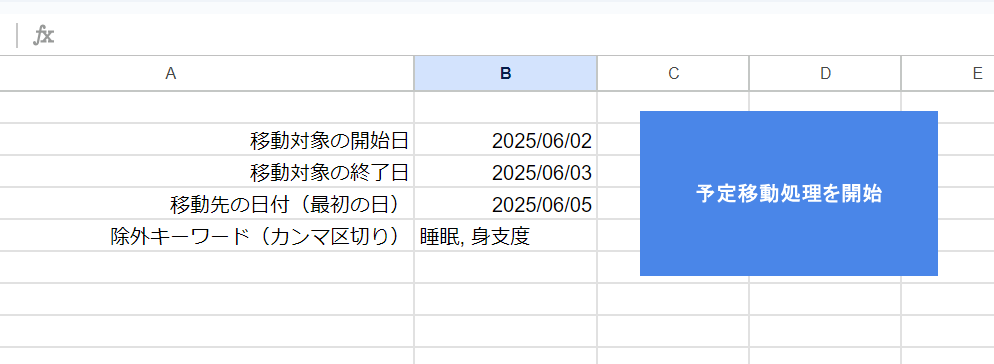
ここで1つポイントとして、取得した移動対象の終了日(endDateValue)は「セルに入力されている日付の00:00 = 一日の始まり」となっており、このままだと最終日の予定が正しく取得できない。
そのため、25~26行目の処理を行い終了日の日付に+1日することで、後続の処理で最終日の予定も正しく取得できるようにしている。
また、移動対象の開始日(startDateValue)と移動先の日付(targetDateValue)の差も後続の処理で使用するため、このタイミングで出しておく。
最後に取得した4つの値をオブジェクトの形式で返し、変数settingsに格納する。
fetchEvents 関数
/**
* 指定期間内の予定を取得する(除外キーワードを含む予定は除外)
* @param {Date} startDate - 開始日
* @param {Date} endDate - 終了日
* @param {Array} excludeKeywords - 除外キーワードのリスト
* @return {Array} 取得した予定の配列
*/
function fetchEvents(startDate, endDate, excludeKeywords) {
// デフォルトカレンダーを取得
const calendar = CalendarApp.getDefaultCalendar();
// 指定期間の予定を取得
const allEvents = calendar.getEvents(startDate, endDate);
// 除外キーワードを含まない予定だけをフィルタリング
const filteredEvents = allEvents.filter((event) => {
const title = event.getTitle();
return !excludeKeywords.some((keyword) => title.includes(keyword));
});
return filteredEvents;
}
getSettings 関数で取得した各情報をもとに、移動対象の予定を取得する関数。
まず最初に、操作対象のGoogleカレンダー自体を取得する。ここでは「getDefaultCalendar()」としてデフォルトカレンダー(Googleカレンダーを開いて一番最初に表示されるもの、大抵は自身のユーザアカウントのカレンダー)を取得しているが、明確に「○○のカレンダーを取得したい」という場合には「getCalendarById()」などで一意に指定することも可能だ。
続いて指定期間の予定をすべて取得したあと、「filter()」メソッドで各予定の除外判定をしていく。少し書き方が複雑なように見えるが、ここでは
とすることで、「除外キーワードを "含まない" 予定」に対してのみ "true" を返し、最終的に移動対象の予定(filteredEvents)として格納している。
moveEvents 関数
/**
* 予定を指定した日数分移動する
* @param {Array} events - 移動する予定の配列
* @param {number} daysDifference - 移動する日数
* @return {Object} 移動結果の統計
*/
function moveEvents(events, daysDifference) {
let movedCount = 0;
// 各予定を移動
events.forEach((event) => {
// 開始時刻と終了時刻を取得
const startTime = event.getStartTime();
const endTime = event.getEndTime();
// 新しい日時を計算(時刻はそのまま、日付だけ移動)
const newStartTime = new Date(startTime);
newStartTime.setDate(startTime.getDate() + daysDifference);
const newEndTime = new Date(endTime);
newEndTime.setDate(endTime.getDate() + daysDifference);
// 予定を移動
event.setTime(newStartTime, newEndTime);
movedCount++;
});
return movedCount;
}
実際にGoogleカレンダー上の予定の移動操作を行う関数で、引数には前段までの処理で取得した移動対象の予定(events)と日数差(daysDifference)が渡される。
まず各予定に対して1つずつ「forEach()」文で処理を回していく。コード内のコメントにも記載しているが、ループ内の処理の流れとしては以下の通り。
・予定の開始時刻(startTime)と終了時刻(endTime)を取得
・startTimeに対してnewStartTimeという名前で新しくDateオブジェクトを作成し、「setDate()」メソッドで元の予定の日付に日数差を足し合わせることで移動先の日付を設定
・最後に「setTime()」メソッドで予定の新しい日付をミリ秒単位で設定
これで各予定に新しい日付(=移動先の日付)が設定され、結果的に予定が移動されたことになる。
またメイン関数での最後のログ出力用に、処理が行われるごとにカウンタ用の変数(movedCount)をインクリメントしておき、すべての処理が終了後に値をメイン関数に返している。
まとめ
今回は「指定された期間のGoogleカレンダー上の予定を別日に移動させるスクリプト」について、GASのコードの紹介と各処理の簡単な解説をした。
Googleカンレンダーの操作というと何となく難しく聞こえるが、シンプルな処理の組み合わせで意外と簡単に実現できてしまうものなので、興味がある方はぜひ自身のカレンダーに対して使ってみて欲しい。